While writing code much time we need to use Loop, and all languages provide Loop.o.e., For Loop, Do while loop, etc.
I assume you all aware of using the loop that it offers a quick and easy way to do something repeatedly. And For loop repeats until a specified condition evaluates to false.
5 simple ways we use jQuery each() method.
- To iterate over an Array (Foreach loop).
- To iterate over an Array of Object.
- To iterate over HTML element (LI tag).
- To iterate over Table Row (tr) and get TD value.
- To iterate over Complex JSON Data.
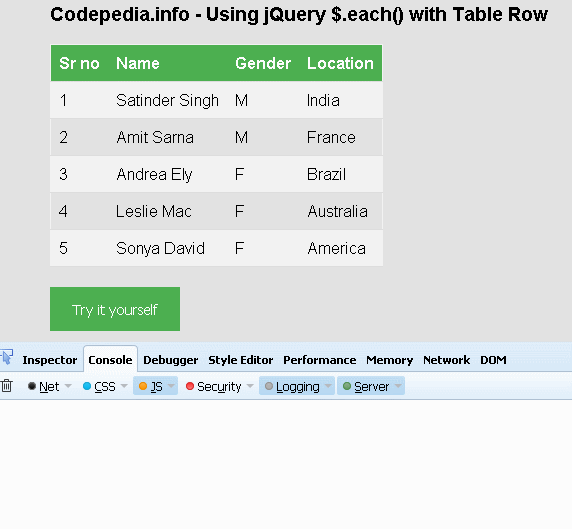
#1 Use jQuery each() function to iterate over an Array.
Let's have an array object with some values in it. As you see below myArray is an array variable that holds values as some fruits name.
Now we want to display all fruit names one by one i.e., using jQuery each function we can iterate over this array variable, and display all the item values.
jQuery Code:
// here's my array variable
var myArray = ["apple", "mango", "orange", "grapes", "banana"];
$.each(myArray, function (index, value) {
console.log(index+' : '+value);
});
Output: So here is the output which displays index and values, by a loop through arrays.
#2 To iterate over an Array of Objects.
Let us take another variable i.e., an array of objects, with some data.
Now using jQuery .each() methods, we make an iterate over this JS object and display its records.
jQuery Code:
var obj= [
{ FirstName: "Andrea" , LastName:"Ely", Gender:"F"},
{ FirstName: "John" , LastName:"Shaw", Gender:"M"},
{ FirstName: "Leslie" , LastName:"Mac", Gender:"F"},
{ FirstName: "David" , LastName:"Millr", Gender:"M"},
{ FirstName: "Rehana" , LastName:"Rewa", Gender:"F"}
]
$.each(obj, function (index, value) {
var first_name=value.FirstName;
var last_name=value.LastName;
console.log(first_name+" "+last_name);
});
OUTPUT: Here's the output, that shows FirstName and LastName from the object variable.
#3 To iterate over a list element.
Here in this example will loop over the HTML element .i.e LI tag and display the text of the li tag. Our HTML markup UL, Li tag is written below.
Now again we are going to use jquery .each() which will loop over the Li tag and get the text of each LI tag and display its text.
HTML Markup:
<ul id="ul_Items">
<li class='fruits' >Apple</li>
<li class='fruits' >Mango</li>
<li class='automobile' >Honda Accord</li>
<li class='automobile' >Harley Davidson</li>
<li class='fruits' >Oranges</li>
<li class='fruits' >Grapes</li>
<li class='automobile' >Royal Enfield</li>
</ul>
jQuery Code:
$("#ul_Items li").each(function(){
var self=$(this);
console.log(self.text());
});
Another way we can achieve the same result i.e., as written below
$.each($("#ul_Items li"),function(){
var self=$(this);
console.log(self.text());
});
Output:
Finally, we were able to display the text of all li tags.
#4 To iterate over Table Row [ TR ].
Here we had an HTML table and added some dummy rows. Now we want to read each table cell value. In jQuery, each table row tr data get fetch, by making a foreach loop in the HTML table. For that we use built-in $.each() method i.e using jQuery each tr row data gets read.
Now using the $.each() method, we fetch every table row cell value. i.e. each td value.
<table id="myTable" style="margin-left:50px;">
<tr><th>Sr no</th><th>Name</th><th>Gender</th><th>Location</th></tr>
<tr><td> 1</td><td>Satinder Singh</td><td> M </td><td> India </td></tr>
<tr><td> 2 </td><td>Amit Sarna</td><td> M </td><td> France </td></tr>
<tr><td> 3 </td><td>Andrea Ely</td><td> F </td><td> Brazil </td></tr>
<tr><td> 4 </td><td>Leslie Mac </td><td> F </td><td> Australia </td></tr>
<tr><td> 5 </td><td>Sonya David </td><td> F </td><td>America</td></tr>
</table>
jQuery Code:
$(".button").on('click', function () {
$("#myTable tr").each(function () {
var self = $(this);
var col_1_value = self.find("td:eq(0)").text().trim();
var col_2_value = self.find("td:eq(1)").text().trim();
var col_3_value = self.find("td:eq(2)").text().trim();
var col_4_value = self.find("td:eq(3)").text().trim();
var result = col_1_value + " - " + col_2_value + " - " + col_3_value + " - " + col_4_value;
console.log(result);
});
});
Here are we able to loop over the HTML table row and fetch the td value.
#5 To iterate over Complex JSON Data
Now we are going to iterate over complex JSON data. While working with complex data we need to work with nested .each() to get respective data.
Here is our JSON data which has records as company name, and its multiple model id, model name respective.
What we have to do is, we want to display all company names with respected models' names on the table.
JSON DATA:
var json = [{"Company_ID":"101",
"Company_Name":"iPhone",
"Models":[
{"Model_ID":"101-M1","Model_Name":"iPhone SE"},
{"Model_ID":"101-M2","Model_Name":"iPhone 11"},
{"Model_ID":"101-M3","Model_Name":"iPhone X Pro"}]
},
{"Company_ID":"201",
"Company_Name":"Vivo",
"Models":[
{"Model_ID":"201-M1","Model_Name":"Vivo Z1X Pro"},
{"Model_ID":"201-M2","Model_Name":"Vivo 17 Pro"},
{"Model_ID":"201-M3","Model_Name":"Vivo UD"}]
}];
HTML Markup:
<table id="myTable" class="table">
<tr>
<th>Company Name</th>
<th>Models</th>
</tr>
</table>
Here we added a table tag on our webpage, which we use to display our JSON data. So here we are going to display the company name and its model's name.
jQuery Code: Use of nested .each() method
var fragment="";
var modelHTML="";
$.each(json, function (index, value) {
// now we have company name and its now,
var Company_ID=value.Company_ID;
var Company_ID=value.Company_Name;
//now we do nested loop to get its models details.
var objModel=value.Models;
modelHTML +="";
$.each(objModel, function (ind, val) {
var Model_ID=val.Model_ID;
var Model_Name=val.Model_Name;
modelHTML +="<li>"+Model_Name+"</li>";
});
modelHTML +="<ul>";
fragment +="<tr><td>"+Company_ID+"</td> <td>"+modelHTML+" </td></tr>";
modelHTML="";
});
$("#myTable").append(fragment);
Here in variable modelHTML , where add all the model's detail, which later append in the variable fragment. Finally, we append it to myTable which is our table element.
BONUS: Let's make it more practical real programming scenario, now what if a user wants to display only fruits. i.e., show only particular LI text whose text are fruits.
If you notice in our HTML markup, we have added a class name for each li tag .i.e fruits and automobile respectively. Now using this class, we can filter our Li tags. Let's see here now what we want to display.
jQuery Code:
$("#ul_Items li").each(function(){
var self=$(this);
// this will check if li has class fruits then only will display.
if(self.hasClass("fruits"))
{
console.log(self.text());
}else
{
// other code logic
}
});
Another way is if you were sure to show only LI tag with having the text as fruits. Then the best way is to select only those Li tags and loop over them, rather than loop over all Li tags which has shown in the above example.
So now jquery code to loop over li tag having class as fruits look like as written below.
jQuery Code: To display li tag having the text as fruits
$("#ul_Items li.fruits").each(function(){
var self=$(this);
console.log(self.text());
});
OUTPUT:
Conclusion: Here in this article we learn 5 different ways of how to use jQuery each() method with a live example. In short, if we want to loop over a simple array, complex JSON object, or over HTML tag, i.e., loop over table row tr or list tag, then using each() method we can achieve all.
For Reference:
You can also check these articles:
- Preview Image before uploads it with jQuery in Asp.net.
- How to get the filename, size, type count in jQuery [input file, File Api].
- Create a dynamic HTML table using jquery.
- How to remove table row tr in jquery on button click.
- Jquery: How to disable Button, Div, Anchor tag.
Thank you for reading, pls keep visiting this blog and share this in your network. Also, I would love to hear your opinions down in the comments.
PS: If you found this content valuable and want to thank me? 👳 Buy Me a Coffee
Post Comment
Your email address will not be published. Required fields are marked *