Overview: Here in this article will see asp.net jQuery Ajax JSON example, i.e. calling jQuery Ajax WebMethod and get JSON format result (JSON data) in Asp.net C# Webform. In short a simple jQuery Ajax JSON example in Asp.net C# with MS SQL Server database.
If you are looking for how to get JSON formatted results from jquery ajax call in asp.net C#, then this post will explain step by step process. You can also check 3 ways to convert DataTable into JSON String in Asp.net C#.
What is jQuery AJAX?
AJAX stands for Asynchronous JavaScript and XML and using ajax we load data from the server without reloading the whole web page refresh. i.e we can update a specific part of our web page dynamically without page refresh. By using jQuery AJAX methods, we can request HTML, XML, or JSON from the server using both HTTP Get and HTTP Post requests.
Am assuming you are aware of Asp.net C# Webservice, i.e., (ASMX file) Webservice.ASMX, WebMethods, and familiar with jQuery Ajax syntax.
And it's very useful if you want to save or insert data into the database without page postback or page refresh .i.e Insert data into the database using jQuery Ajax.
In this tutorial, we make a jQuery Ajax call and in response will get the JSON data, i.e., here we get a list of all cars and other detailed information from our database ( MS SQL server) via WebMethod (ASMX file).
Steps to create jQuery Ajax JSON example in Asp.net C#.
- Download the jQuery library and add Html Markup.
- Calling jQuery Ajax method.
- C#: Create Class and WebMethod, which returns JSON Object.
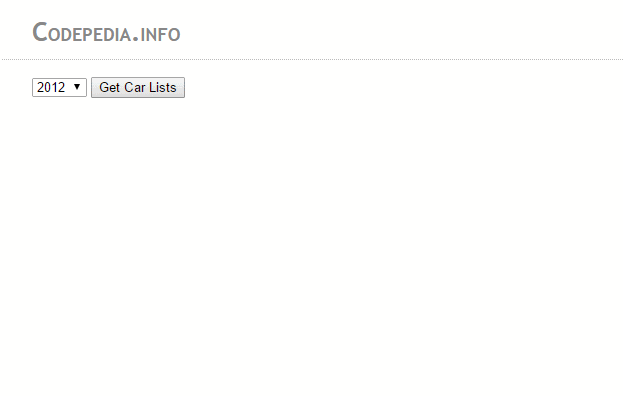

# Download the jQuery library and add Html Markup.
First, we open Visual Studio IDE, create a new project, and then we added a new Asp.net web page and named as myJsonTuts.aspx. On our Asp.net web page, we add a button control and import jQuery library.
You can download jQuery files from jQuery.com, or you can use Google-hosted files. So now our HTML markup looks like as shown below.
<head>
<script src="latestJs_1.11/jquery.min.js"></script>
</head>
<body>
<form runat="server">
<div>
<select id="ddlSelectYear">
<option>2014</option>
<option>2015</option>
</select>
<button id="myButton">Get Car Lists</button>
<div id="contentHolder"></div>
</div>
</form>
</body>
# Client-Side: Calling jQuery Ajax method.
Now we write client-side code .i.e. on button click we make a jQuery Ajax call with returns JSON data, check this for dynamic button click event in jQuery.
Here in our case, we fetch the list of cars along with other information from the database MS SQL server via C# WebMethod, which we create later on the server-side.
Let's first check how our Client-side code looks as shown below
//*
$("#myButton").on("click", function (e) {
e.preventDefault();
var aData= [];
aData[0] = $("#ddlSelectYear").val();
$("#contentHolder").empty();
var jsonData = JSON.stringify({ aData:aData});
$.ajax({
type: "POST",
//getListOfCars is my webmethod
url: "WebService.asmx/getListOfCars",
data: jsonData,
contentType: "application/json; charset=utf-8",
dataType: "json", // dataType is json format
success: OnSuccess,
error: OnErrorCall
});
function OnSuccess(response) {
console.log(response.d)
}
function OnErrorCall(response) { console.log(error); }
});
//*
Here in the above code on our button click, we make an ajax call.
You may notice we have set dataType="JSON" that is because we want our ajax response in JSON format.
# Creating a class and WebMethod, which returns list collection as JSON data.
Now we add a Webservice file (ASMX file) in our project, here we added a file and named it as myfunction.asmx. In our Webservice (.asmx file) we also created a class .i.e Cars which has properties as carName, carRating, carYear.
Now we write a WebMethod as we want to get the list of all cars, here getListofCars is our WebMethod, which fires the select query to the database, pulls the record from the database, and adds it to our class object Car.
In response, we return this Car class object.
//Created a class
public class Cars
{
public string carName;
public string carRating;
public string carYear;
}
Now we write our WebMethod, which returns a List of Cars details.[WebMethod]
public List<Cars> getListOfCars(List<string> aData)
{
SqlDataReader dr;
List<Cars> carList = new List<Cars>();
using (SqlConnection con = new SqlConnection(conn))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.CommandText = "spGetCars";
cmd.CommandType = CommandType.StoredProcedure;
cmd.Connection = con;
cmd.Parameters.AddWithValue("@makeYear", aData[0]);
con.Open();
dr = cmd.ExecuteReader(CommandBehavior.CloseConnection);
if (dr.HasRows)
{
while (dr.Read())
{
string carname = dr["carName"].ToString();
string carrating = dr["carRating"].ToString();
string makingyear = dr["carYear"].ToString();
carList.Add(new Cars
{
carName = carname,
carRating = carrating,
carYear = makingyear
});
}
}
}
}
return carList;
}
In response, we will get data in JSON format, check this in your browser console, the JSON format list of cars is available as shown above in Fig 1.
function OnSuccess(response.d)) {
console.log(response.d)
}
Note: Always use response.d to get the jquery ajax response in Asp.net C#.
Now we write some more code on our client-side .i.e how to display JSON data list of the car on our Web page.
In short, we have to write some jQuery code to handle the response `JSON Data`. So now our success function looks like as shown below.
function OnSuccess(response) {
var items = response.d;
var fragment="<ul>"
$.each(items, function (index, val) {
var carName = val.carName;
var carRating = val.carRating;
var carYear = val.carYear;
fragment += "<li> "+carName+" :: "+carRating+" - "+carYear+"</li>";
});
$("#contentHolder").append(fragment);
}
Here we stored the response in a variable item and then using the jQuery $.each() method we make a loop over data, get and set the value to '<li>' tag, and append to parent `<UL>`
# OutPut: Asp.net ajax JSON example:
Finally, our Output which displays the list of car and other information.
Conclusion: In this article, we learn how to make a jquery ajax call in Asp.net C# .ie using WebMethod ( .asmx file) and returns JSON format data. Also, we display this JSON data into our HTML .i.e onSuccess method we create a List of Cars with other information ( Li tag) using JSON data.
You must also check these articles:- How to send JSON data to Generic Handler ashx file in Asp.net.
- Chart.js + Asp.net: Create Pie chart with JSON response in Jquery.
- An easy way to upload Bulk Image in Asp.net C# using Dropzone JS.
Other Reference:
Thank you for reading, pls keep visiting this blog and share this in your network. Also, I would love to hear your opinions down in the comments.
PS: If you found this content valuable and want to thank me? 👳 Buy Me a Coffee
Post Comment
Your email address will not be published. Required fields are marked *